Assembly Gui Programming
Question or issue on macOS:
GUI is managed by the operating system, and components of GUI are also created by the operating systems. So just call OS GUI functions from assembly. There’s nothing magical about it, and it is no different from writing a GUI application in C. It is actually quite easy to find ASM GUI application examples. This feature is not really that important in programming assembly because you can write your codes in the notepad or any text editor applications. Add Tip Ask Question Comment Download 1 Person Made This Project! Assembly language is a low-level programming language for niche platforms such as IoTs, device drivers, and embedded systems. Usually, it’s the sort of language that Computer Science students should cover in their coursework and rarely use in their future jobs.
I’d like to know how can I do a simple assembly program for Mac OS X that shows a window on the screen and put some coloured text on that window. The code may call some Carbon or Cocoa APIs. I need some code for the nasm sintaxe.
- Turbo Assembler (sometimes shortened to the name of the executable, TASM) is an assembler for software development published by Borland in 1989. It runs on and produces code for 16- or 32-bit x86 MS-DOS and compatibles or Microsoft Windows.
- Assembly GUI programming for Mac OS X. December 16, 2020 Abreonia Ng. Question or issue on macOS: I’d like to know how can I do a simple assembly program for.
I saw in http://snipplr.com/view/29150/assembly-code-nasm-for-mac–hello-world the next code that works fine, but it´s not graphic.
What Is Gui Programming
Thanks for any help
How to solve this problem?
Solution no. 1:
Assembly Gui Programming Tutorial
This is not Carbon as requested in the comments in the previous answers, but it may help you get a step further ahead in your noble pursuit:
Solution no. 2:
You can call Carbon APIs with call
like this:
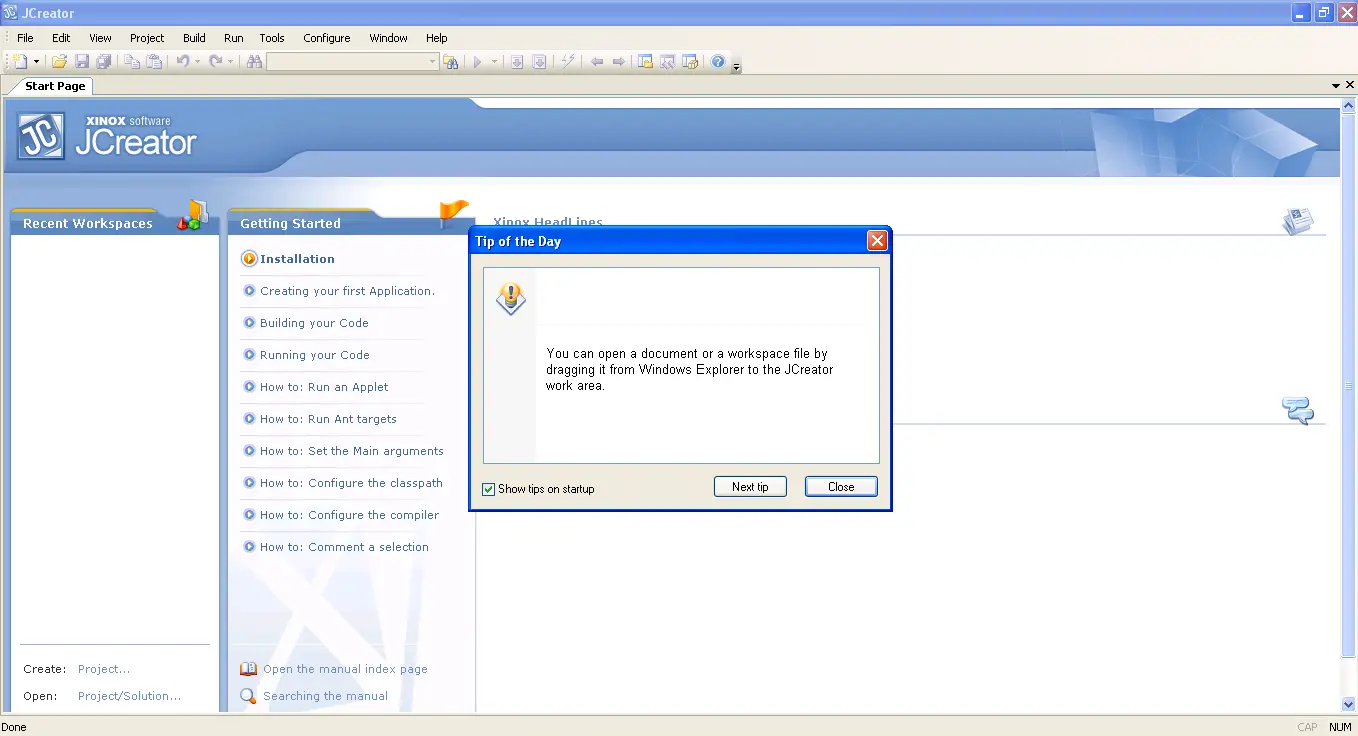
You can pass arguments also, but I’m unsure how to do that. Probably push
ed onto the stack in reversed order just before the call
:
You can look in how you C code compiles into assembly, like this:
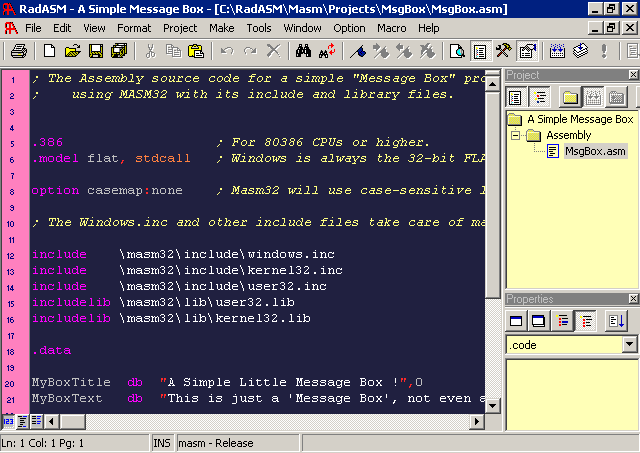
Hope this helps!
Welcome!This area on Webster is dedicated to Win32 assembly language programming topics. Specifically, this section deals with the Win32 API, HOWL (the HLA Object Windows Library), and GUI-based programming in assembly language. | ||||
Windows Programming in Assembly Language | ||||
Several years ago, Randy Hyde began translating Charles Peztold's 'Windows Programming' into assembly language. This book teaches Win32 GUI application programming using the Win32 API. For copyright reasons, plus the fact that the material was just not the right way to write Win32 assembly code, Randy abandoned that project. However, the first several chapters are available on-line and still provide a great reference for programmers who want to write low-level Win32 code. Preview the PDF or HTML versions of this new book by clicking on the links to the right. | ||||
HOWL (HLA Object Windows Library) has arrived! HOWL makes Win32 assembly language programming easier than ever before. By providing an 'Application Framework' (much like Microsoft's MFC or Borland's VCL), HOWL takes care of all the grunt work required by low-level Win32 API programming and lets you concentrate on writing your applications. This is the right way to write Win32 GUI applications in assembly language. Note that the tutorial source code is available as part of the HLA Examples download (get it here). If you're going to work through these tutorials, you should download the entire HLA examples zip file. You'll find the HOWL examples in the win32howl subdirectory. Also note that the HOWL documentation is part of the HLA Standard Library documentation. You can find the HOWL documentation here. The example source files to the right generally appear in pairs: a 'standard' version and an 'x_*' version. The standard version uses the HOWL Declarative Language (HDL) to define all the widgets (controls) on a form (window). Those with the 'x_' prefix eschew the HDL and make manual calls to the HOWL library code. The tutorials mainly discuss the standard versions of these sample programs; those who are interested in seeing the lower-level implementation of the code might want to take a peek at the 'x_*' versions of these applications. 1:Hello World 2: Buttons 3: Check Boxes 4: Radio Buttons 5: Labels 6: Menus 7: Graphic Objects This tutorial teaches you how to draw various graphic objects on a form including rectangles, round rectangles, pie wedges, ellipses and circles, and polygons. This tutorial also teaches you how to overlay various graphic objects using a transparent background color and how to create clickable graphics objects. It demonstrates how to move, resize, show/hide, and change the color of these graphic objects. | 1: Hello World | |||
tutorial | ||||
helloworld.hla | ||||
x_helloworld.hla | ||||
2: Buttons | ||||
tutorial | ||||
button1.hla | ||||
x_button1.hla | ||||
button2.hla | ||||
x_button2.hla | ||||
button3.hla | ||||
x_button3.hla | ||||
3: Check Boxes | ||||
tutorial | ||||
checkbox1.hla | ||||
x_checkbox1.hla | ||||
checkbox2.hla | ||||
x_checkbox2.hla | ||||
checkbox3.hla | ||||
x_checkbox3.hla | ||||
checkbox4.hla | ||||
x_checkbox4.hla | ||||
4: Radio Buttons | ||||
tutorial | ||||
radioSet1.hla | ||||
x_radioset1.hla | ||||
radioset2.hla | ||||
x_radioset2.hla | ||||
radioset3.hla | ||||
x_radioset3.hla | ||||
5: Labels | ||||
tutorial | ||||
label1.hla | ||||
x_label1.hla | ||||
label2.hla | ||||
x_label2.hla | ||||
6: Menus | ||||
tutorial | ||||
menu1.hla | ||||
x_menu1.hla | ||||
7: Graphic Objects | ||||
tutorial | ||||
graphics1.hla | ||||
x_graphics1.hla | ||||
graphics2.hla | ||||
x_graphics2.hla | ||||
graphics3.hla | ||||
x_graphics3.hla | ||||
graphics4.hla | ||||
x_graphics4.hla | ||||
graphics5.hla | ||||
x_graphics5.hla |
Win32 API Documentation Project | ||
Got Ink? |
The following are some of the reknown Iczelion win32 tutorials with their text and code updated for HLA (the original tutorials used MASM). Check back here frequently because new translations will appear on a regular basis. |
